Gtk.Image¶
Example¶
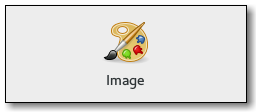
- Subclasses:
None
Methods¶
- Inherited:
Gtk.Misc (4), Gtk.Widget (278), GObject.Object (37), Gtk.Buildable (10)
- Structs:
class |
|
class |
|
class |
|
class |
|
class |
|
class |
|
class |
|
class |
|
class |
|
class |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Virtual Methods¶
- Inherited:
Properties¶
- Inherited:
Name |
Type |
Flags |
Short Description |
---|---|---|---|
r/w |
Filename to load and display |
||
r/w |
The |
||
r/w |
The name of the icon from the icon theme |
||
d/r/w |
Icon set to display |
||
r/w/en |
Symbolic size to use for stock icon, icon set or named icon |
||
r/w |
A |
||
r/w |
|
||
r/w/en |
Pixel size to use for named icon |
||
r/w |
The resource path being displayed |
||
d/r/w |
Stock ID for a stock image to display |
||
r |
The representation being used for image data |
||
r/w |
A |
||
r/w/en |
Whether to use icon names fallback |
Style Properties¶
- Inherited:
Signals¶
- Inherited:
Fields¶
- Inherited:
Name |
Type |
Access |
Description |
---|---|---|---|
misc |
r |
Class Details¶
- class Gtk.Image(**kwargs)¶
- Bases:
- Abstract:
No
- Structure:
The
Gtk.Image
widget displays an image. Various kinds of object can be displayed as an image; most typically, you would load aGdkPixbuf.Pixbuf
(“pixel buffer”) from a file, and then display that. There’s a convenience function to do this,Gtk.Image.new_from_file
(), used as follows:GtkWidget *image; image = gtk_image_new_from_file ("myfile.png");
If the file isn’t loaded successfully, the image will contain a “broken image” icon similar to that used in many web browsers. If you want to handle errors in loading the file yourself, for example by displaying an error message, then load the image with
GdkPixbuf.Pixbuf.new_from_file
(), then create theGtk.Image
withGtk.Image.new_from_pixbuf
().The image file may contain an animation, if so the
Gtk.Image
will display an animation (GdkPixbuf.PixbufAnimation
) instead of a static image.Gtk.Image
is a subclass ofGtk.Misc
, which implies that you can align it (center, left, right) and add padding to it, usingGtk.Misc
methods.Gtk.Image
is a “no window” widget (has noGdk.Window
of its own), so by default does not receive events. If you want to receive events on the image, such as button clicks, place the image inside aGtk.EventBox
, then connect to the event signals on the event box.- Handling button press events on a
Gtk.Image
.
static gboolean button_press_callback (GtkWidget *event_box, GdkEventButton *event, gpointer data) { g_print ("Event box clicked at coordinates %f,%f\n", event->x, event->y); // Returning TRUE means we handled the event, so the signal // emission should be stopped (don’t call any further callbacks // that may be connected). Return FALSE to continue invoking callbacks. return TRUE; } static GtkWidget* create_image (void) { GtkWidget *image; GtkWidget *event_box; image = gtk_image_new_from_file ("myfile.png"); event_box = gtk_event_box_new (); gtk_container_add (GTK_CONTAINER (event_box), image); g_signal_connect (G_OBJECT (event_box), "button_press_event", G_CALLBACK (button_press_callback), image); return image; }
When handling events on the event box, keep in mind that coordinates in the image may be different from event box coordinates due to the alignment and padding settings on the image (see
Gtk.Misc
). The simplest way to solve this is to set the alignment to 0.0 (left/top), and set the padding to zero. Then the origin of the image will be the same as the origin of the event box.Sometimes an application will want to avoid depending on external data files, such as image files. GTK+ comes with a program to avoid this, called “gdk-pixbuf-csource”. This library allows you to convert an image into a C variable declaration, which can then be loaded into a
GdkPixbuf.Pixbuf
usingGdkPixbuf.Pixbuf.new_from_inline
().- CSS nodes
Gtk.Image
has a single CSS node with the name image. The style classes may appear on image CSS nodes: .icon-dropshadow, .lowres-icon.- classmethod new()[source]¶
- Returns:
a newly created
Gtk.Image
widget.- Return type:
Creates a new empty
Gtk.Image
widget.
- classmethod new_from_animation(animation)[source]¶
- Parameters:
animation (
GdkPixbuf.PixbufAnimation
) – an animation- Returns:
a new
Gtk.Image
widget- Return type:
Creates a
Gtk.Image
displaying the given animation. TheGtk.Image
does not assume a reference to the animation; you still need to unref it if you own references.Gtk.Image
will add its own reference rather than adopting yours.Note that the animation frames are shown using a timeout with
GLib.PRIORITY_DEFAULT
. When using animations to indicate busyness, keep in mind that the animation will only be shown if the main loop is not busy with something that has a higher priority.
- classmethod new_from_file(filename)[source]¶
-
Creates a new
Gtk.Image
displaying the file filename. If the file isn’t found or can’t be loaded, the resultingGtk.Image
will display a “broken image” icon. This function never returnsNone
, it always returns a validGtk.Image
widget.If the file contains an animation, the image will contain an animation.
If you need to detect failures to load the file, use
GdkPixbuf.Pixbuf.new_from_file
() to load the file yourself, then create theGtk.Image
from the pixbuf. (Or for animations, useGdkPixbuf.PixbufAnimation.new_from_file
()).The storage type (
Gtk.Image.get_storage_type
()) of the returned image is not defined, it will be whatever is appropriate for displaying the file.
- classmethod new_from_gicon(icon, size)[source]¶
- Parameters:
icon (
Gio.Icon
) – an iconsize (
int
) – a stock icon size (Gtk.IconSize
)
- Returns:
a new
Gtk.Image
displaying the themed icon- Return type:
Creates a
Gtk.Image
displaying an icon from the current icon theme. If the icon name isn’t known, a “broken image” icon will be displayed instead. If the current icon theme is changed, the icon will be updated appropriately.New in version 2.14.
- classmethod new_from_icon_name(icon_name, size)[source]¶
- Parameters:
size (
int
) – a stock icon size (Gtk.IconSize
)
- Returns:
a new
Gtk.Image
displaying the themed icon- Return type:
Creates a
Gtk.Image
displaying an icon from the current icon theme. If the icon name isn’t known, a “broken image” icon will be displayed instead. If the current icon theme is changed, the icon will be updated appropriately.New in version 2.6.
- classmethod new_from_icon_set(icon_set, size)[source]¶
- Parameters:
icon_set (
Gtk.IconSet
) – aGtk.IconSet
size (
int
) – a stock icon size (Gtk.IconSize
)
- Returns:
a new
Gtk.Image
- Return type:
Creates a
Gtk.Image
displaying an icon set. Sample stock sizes areGtk.IconSize.MENU
,Gtk.IconSize.SMALL_TOOLBAR
. Instead of using this function, usually it’s better to create aGtk.IconFactory
, put your icon sets in the icon factory, add the icon factory to the list of default factories withGtk.IconFactory.add_default
(), and then useGtk.Image.new_from_stock
(). This will allow themes to override the icon you ship with your application.The
Gtk.Image
does not assume a reference to the icon set; you still need to unref it if you own references.Gtk.Image
will add its own reference rather than adopting yours.Deprecated since version 3.10: Use
Gtk.Image.new_from_icon_name
() instead.
- classmethod new_from_pixbuf(pixbuf)[source]¶
- Parameters:
pixbuf (
GdkPixbuf.Pixbuf
orNone
) – aGdkPixbuf.Pixbuf
, orNone
- Returns:
a new
Gtk.Image
- Return type:
Creates a new
Gtk.Image
displaying pixbuf. TheGtk.Image
does not assume a reference to the pixbuf; you still need to unref it if you own references.Gtk.Image
will add its own reference rather than adopting yours.Note that this function just creates an
Gtk.Image
from the pixbuf. TheGtk.Image
created will not react to state changes. Should you want that, you should useGtk.Image.new_from_icon_name
().
- classmethod new_from_resource(resource_path)[source]¶
-
Creates a new
Gtk.Image
displaying the resource file resource_path. If the file isn’t found or can’t be loaded, the resultingGtk.Image
will display a “broken image” icon. This function never returnsNone
, it always returns a validGtk.Image
widget.If the file contains an animation, the image will contain an animation.
If you need to detect failures to load the file, use
GdkPixbuf.Pixbuf.new_from_file
() to load the file yourself, then create theGtk.Image
from the pixbuf. (Or for animations, useGdkPixbuf.PixbufAnimation.new_from_file
()).The storage type (
Gtk.Image.get_storage_type
()) of the returned image is not defined, it will be whatever is appropriate for displaying the file.New in version 3.4.
- classmethod new_from_stock(stock_id, size)[source]¶
- Parameters:
stock_id (
str
) – a stock icon namesize (
int
) – a stock icon size (Gtk.IconSize
)
- Returns:
a new
Gtk.Image
displaying the stock icon- Return type:
Creates a
Gtk.Image
displaying a stock icon. Sample stock icon names areGtk.STOCK_OPEN
,Gtk.STOCK_QUIT
. Sample stock sizes areGtk.IconSize.MENU
,Gtk.IconSize.SMALL_TOOLBAR
. If the stock icon name isn’t known, the image will be empty. You can register your own stock icon names, seeGtk.IconFactory.add_default
() andGtk.IconFactory.add
().Deprecated since version 3.10: Use
Gtk.Image.new_from_icon_name
() instead.
- classmethod new_from_surface(surface)[source]¶
- Parameters:
surface (
cairo.Surface
orNone
) – acairo.Surface
, orNone
- Returns:
a new
Gtk.Image
- Return type:
Creates a new
Gtk.Image
displaying surface. TheGtk.Image
does not assume a reference to the surface; you still need to unref it if you own references.Gtk.Image
will add its own reference rather than adopting yours.New in version 3.10.
- get_animation()[source]¶
- Returns:
the displayed animation, or
None
if the image is empty- Return type:
Gets the
GdkPixbuf.PixbufAnimation
being displayed by theGtk.Image
. The storage type of the image must beGtk.ImageType.EMPTY
orGtk.ImageType.ANIMATION
(seeGtk.Image.get_storage_type
()). The caller of this function does not own a reference to the returned animation.
- get_gicon()[source]¶
- Returns:
- gicon:
- size:
place to store an icon size (
Gtk.IconSize
), orNone
- Return type:
Gets the
Gio.Icon
and size being displayed by theGtk.Image
. The storage type of the image must beGtk.ImageType.EMPTY
orGtk.ImageType.GICON
(seeGtk.Image.get_storage_type
()). The caller of this function does not own a reference to the returnedGio.Icon
.New in version 2.14.
- get_icon_name()[source]¶
- Returns:
- icon_name:
place to store an icon name, or
None
- size:
place to store an icon size (
Gtk.IconSize
), orNone
- Return type:
Gets the icon name and size being displayed by the
Gtk.Image
. The storage type of the image must beGtk.ImageType.EMPTY
orGtk.ImageType.ICON_NAME
(seeGtk.Image.get_storage_type
()). The returned string is owned by theGtk.Image
and should not be freed.New in version 2.6.
- get_icon_set()[source]¶
- Returns:
- icon_set:
location to store a
Gtk.IconSet
, orNone
- size:
location to store a stock icon size (
Gtk.IconSize
), orNone
- Return type:
(icon_set:
Gtk.IconSet
, size:int
)
Gets the icon set and size being displayed by the
Gtk.Image
. The storage type of the image must beGtk.ImageType.EMPTY
orGtk.ImageType.ICON_SET
(seeGtk.Image.get_storage_type
()).Deprecated since version 3.10: Use
Gtk.Image.get_icon_name
() instead.
- get_pixbuf()[source]¶
- Returns:
the displayed pixbuf, or
None
if the image is empty- Return type:
Gets the
GdkPixbuf.Pixbuf
being displayed by theGtk.Image
. The storage type of the image must beGtk.ImageType.EMPTY
orGtk.ImageType.PIXBUF
(seeGtk.Image.get_storage_type
()). The caller of this function does not own a reference to the returned pixbuf.
- get_pixel_size()[source]¶
- Returns:
the pixel size used for named icons.
- Return type:
Gets the pixel size used for named icons.
New in version 2.6.
- get_stock()[source]¶
- Returns:
- stock_id:
place to store a stock icon name, or
None
- size:
place to store a stock icon size (
Gtk.IconSize
), orNone
- Return type:
Gets the stock icon name and size being displayed by the
Gtk.Image
. The storage type of the image must beGtk.ImageType.EMPTY
orGtk.ImageType.STOCK
(seeGtk.Image.get_storage_type
()). The returned string is owned by theGtk.Image
and should not be freed.Deprecated since version 3.10: Use
Gtk.Image.get_icon_name
() instead.
- get_storage_type()[source]¶
- Returns:
image representation being used
- Return type:
Gets the type of representation being used by the
Gtk.Image
to store image data. If theGtk.Image
has no image data, the return value will beGtk.ImageType.EMPTY
.
- set_from_animation(animation)[source]¶
- Parameters:
animation (
GdkPixbuf.PixbufAnimation
) – theGdkPixbuf.PixbufAnimation
Causes the
Gtk.Image
to display the given animation (or display nothing, if you set the animation toNone
).
- set_from_file(filename)[source]¶
-
See
Gtk.Image.new_from_file
() for details.
- set_from_gicon(icon, size)[source]¶
- Parameters:
icon (
Gio.Icon
) – an iconsize (
int
) – an icon size (Gtk.IconSize
)
See
Gtk.Image.new_from_gicon
() for details.New in version 2.14.
- set_from_icon_name(icon_name, size)[source]¶
- Parameters:
size (
int
) – an icon size (Gtk.IconSize
)
See
Gtk.Image.new_from_icon_name
() for details.New in version 2.6.
- set_from_icon_set(icon_set, size)[source]¶
- Parameters:
icon_set (
Gtk.IconSet
) – aGtk.IconSet
size (
int
) – a stock icon size (Gtk.IconSize
)
See
Gtk.Image.new_from_icon_set
() for details.Deprecated since version 3.10: Use
Gtk.Image.set_from_icon_name
() instead.
- set_from_pixbuf(pixbuf)[source]¶
- Parameters:
pixbuf (
GdkPixbuf.Pixbuf
orNone
) – aGdkPixbuf.Pixbuf
orNone
See
Gtk.Image.new_from_pixbuf
() for details.
- set_from_resource(resource_path)[source]¶
-
See
Gtk.Image.new_from_resource
() for details.
- set_from_stock(stock_id, size)[source]¶
- Parameters:
stock_id (
str
) – a stock icon namesize (
int
) – a stock icon size (Gtk.IconSize
)
See
Gtk.Image.new_from_stock
() for details.Deprecated since version 3.10: Use
Gtk.Image.set_from_icon_name
() instead.
- set_from_surface(surface)[source]¶
- Parameters:
surface (
cairo.Surface
orNone
) – acairo.Surface
orNone
See
Gtk.Image.new_from_surface
() for details.New in version 3.10.
- set_pixel_size(pixel_size)[source]¶
- Parameters:
pixel_size (
int
) – the new pixel size
Sets the pixel size to use for named icons. If the pixel size is set to a value != -1, it is used instead of the icon size set by
Gtk.Image.set_from_icon_name
().New in version 2.6.
Property Details¶
- Gtk.Image.props.file¶
-
Filename to load and display
- Gtk.Image.props.gicon¶
-
The
Gio.Icon
displayed in theGtk.Image
. For themed icons, If the icon theme is changed, the image will be updated automatically.New in version 2.14.
- Gtk.Image.props.icon_name¶
-
The name of the icon in the icon theme. If the icon theme is changed, the image will be updated automatically.
New in version 2.6.
- Gtk.Image.props.icon_set¶
- Name:
icon-set
- Type:
- Default Value:
- Flags:
Icon set to display
Deprecated since version 3.10: Use
Gtk.Image
:icon-name
instead.
- Gtk.Image.props.icon_size¶
- Name:
icon-size
- Type:
- Default Value:
4
- Flags:
Symbolic size to use for stock icon, icon set or named icon
- Gtk.Image.props.pixbuf¶
- Name:
pixbuf
- Type:
- Default Value:
- Flags:
A
GdkPixbuf.Pixbuf
to display
- Gtk.Image.props.pixbuf_animation¶
- Name:
pixbuf-animation
- Type:
- Default Value:
- Flags:
GdkPixbuf.PixbufAnimation
to display
- Gtk.Image.props.pixel_size¶
- Name:
pixel-size
- Type:
- Default Value:
-1
- Flags:
The “pixel-size” property can be used to specify a fixed size overriding the
Gtk.Image
:icon-size
property for images of typeGtk.ImageType.ICON_NAME
.New in version 2.6.
- Gtk.Image.props.resource¶
-
A path to a resource file to display.
New in version 3.8.
- Gtk.Image.props.stock¶
- Name:
stock
- Type:
- Default Value:
- Flags:
Stock ID for a stock image to display
Deprecated since version 3.10: Use
Gtk.Image
:icon-name
instead.
- Gtk.Image.props.storage_type¶
- Name:
storage-type
- Type:
- Default Value:
- Flags:
The representation being used for image data
- Gtk.Image.props.surface¶
- Name:
surface
- Type:
- Default Value:
- Flags:
A
cairo.Surface
to display
- Gtk.Image.props.use_fallback¶
- Name:
use-fallback
- Type:
- Default Value:
- Flags:
Whether the icon displayed in the
Gtk.Image
will use standard icon names fallback. The value of this property is only relevant for images of typeGtk.ImageType.ICON_NAME
andGtk.ImageType.GICON
.New in version 3.0.