Gtk.ListBox¶
Example¶
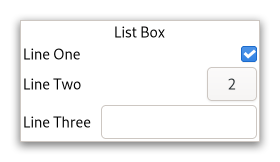
- Subclasses:
None
Methods¶
- Inherited:
Gtk.Widget (183), GObject.Object (37), Gtk.Accessible (17), Gtk.Buildable (1)
- Structs:
class |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Virtual Methods¶
- Inherited:
Gtk.Widget (25), GObject.Object (7), Gtk.Accessible (6), Gtk.Buildable (9)
Properties¶
- Inherited:
Name |
Type |
Flags |
Short Description |
---|---|---|---|
r/w/en |
|||
r/w/en |
|||
r/w/en |
|||
r/w/en |
|||
r/w/en |
Signals¶
- Inherited:
Name |
Short Description |
---|---|
Emitted when the cursor row is activated. |
|
Emitted when the user initiates a cursor movement. |
|
Emitted when a row has been activated by the user. |
|
Emitted when a new row is selected, or (with a |
|
Emitted to select all children of the box, if the selection mode permits it. |
|
Emitted when the set of selected rows changes. |
|
Emitted when the cursor row is toggled. |
|
Emitted to unselect all children of the box, if the selection mode permits it. |
Fields¶
- Inherited:
Class Details¶
- class Gtk.ListBox(**kwargs)¶
- Bases:
- Abstract:
No
Shows a vertical list.
<picture> <source srcset=”list-box-dark.png” media=”(prefers-color-scheme: dark)”> <img alt=”An example
Gtk.ListBox
" src=”list-box.png”> </picture>A
GtkListBox
only containsGtkListBoxRow
children. These rows can by dynamically sorted and filtered, and headers can be added dynamically depending on the row content. It also allows keyboard and mouse navigation and selection like a typical list.Using
GtkListBox
is often an alternative toGtkTreeView
, especially when the list contents has a more complicated layout than what is allowed by aGtkCellRenderer
, or when the contents is interactive (i.e. has a button in it).Although a
GtkListBox
must have onlyGtkListBoxRow
children, you can add any kind of widget to it via [method`Gtk`.ListBox.prepend], [method`Gtk`.ListBox.append] and [method`Gtk`.ListBox.insert] and aGtkListBoxRow
widget will automatically be inserted between the list and the widget.GtkListBoxRows
can be marked as activatable or selectable. If a row is activatable, [signal`Gtk`.ListBox::row-activated] will be emitted for it when the user tries to activate it. If it is selectable, the row will be marked as selected when the user tries to select it.The
GtkListBox
implementation of theGtkBuildable
interface supports setting a child as the placeholder by specifying “placeholder” as the “type” attribute of a<child>
element. See [method`Gtk`.ListBox.set_placeholder] for info.- Shortcuts and Gestures
The following signals have default keybindings:
[signal`Gtk`.ListBox::move-cursor]
[signal`Gtk`.ListBox::select-all]
[signal`Gtk`.ListBox::toggle-cursor-row]
[signal`Gtk`.ListBox::unselect-all]
- CSS nodes
`` list[.separators][.rich-list][.navigation-sidebar][.boxed-list] ╰── row[.activatable] ``
GtkListBox
uses a single CSS node named list. It may carry the .separators style class, when the [property`Gtk`.ListBox:show-separators] property is set. EachGtkListBoxRow
uses a single CSS node named row. The row nodes get the .activatable style class added when appropriate.It may also carry the .boxed-list style class. In this case, the list will be automatically surrounded by a frame and have separators.
The main list node may also carry style classes to select the style of list presentation : .rich-list, .navigation-sidebar or .data-table.
- Accessibility
GtkListBox
uses the [enum`Gtk`.AccessibleRole.list] role andGtkListBoxRow
uses the [enum`Gtk`.AccessibleRole.list_item] role.- classmethod new()[source]¶
- Returns:
a new
GtkListBox
- Return type:
Creates a new
GtkListBox
container.
- append(child)[source]¶
- Parameters:
child (
Gtk.Widget
) – theGtkWidget
to add
Append a widget to the list.
If a sort function is set, the widget will actually be inserted at the calculated position.
- bind_model(model, create_widget_func, *user_data)[source]¶
- Parameters:
model (
Gio.ListModel
orNone
) – theGListModel
to be bound to selfcreate_widget_func (
Gtk.ListBoxCreateWidgetFunc
orNone
) – a function that creates widgets for items orNone
in case you also passedNone
as modeluser_data (
object
orNone
) – user data passed to create_widget_func
Binds model to self.
If self was already bound to a model, that previous binding is destroyed.
The contents of self are cleared and then filled with widgets that represent items from model. self is updated whenever model changes. If model is
None
, self is left empty.It is undefined to add or remove widgets directly (for example, with [method`Gtk`.ListBox.insert]) while self is bound to a model.
Note that using a model is incompatible with the filtering and sorting functionality in
GtkListBox
. When using a model, filtering and sorting should be implemented by the model.
- drag_highlight_row(row)[source]¶
- Parameters:
row (
Gtk.ListBoxRow
) – aGtkListBoxRow
Add a drag highlight to a row.
This is a helper function for implementing DnD onto a
GtkListBox
. The passed in row will be highlighted by setting theGtk.StateFlags.DROP_ACTIVE
state and any previously highlighted row will be unhighlighted.The row will also be unhighlighted when the widget gets a drag leave event.
- drag_unhighlight_row()[source]¶
If a row has previously been highlighted via
Gtk.ListBox.drag_highlight_row
(), it will have the highlight removed.
- get_adjustment()[source]¶
- Returns:
the adjustment
- Return type:
Gets the adjustment (if any) that the widget uses to for vertical scrolling.
- get_row_at_index(index_)[source]¶
- Parameters:
index (
int
) – the index of the row- Returns:
the child
GtkWidget
- Return type:
Gets the n-th child in the list (not counting headers).
If index_ is negative or larger than the number of items in the list,
None
is returned.
- get_row_at_y(y)[source]¶
- Parameters:
y (
int
) – position- Returns:
the row
- Return type:
Gets the row at the y position.
- get_selected_row()[source]¶
- Returns:
the selected row
- Return type:
Gets the selected row, or
None
if no rows are selected.Note that the box may allow multiple selection, in which case you should use [method`Gtk`.ListBox.selected_foreach] to find all selected rows.
- get_selected_rows()[source]¶
- Returns:
A
GList
containing theGtkWidget
for each selected child. Free with g_list_free() when done.- Return type:
Creates a list of all selected children.
- get_selection_mode()[source]¶
- Returns:
a
GtkSelectionMode
- Return type:
Gets the selection mode of the listbox.
- get_tab_behavior()[source]¶
- Returns:
the tab behavior
- Return type:
Returns the behavior of the <kbd>Tab</kbd> and <kbd>Shift</kbd>+<kbd>Tab</kbd> keys.
New in version 4.18.
- insert(child, position)[source]¶
- Parameters:
child (
Gtk.Widget
) – theGtkWidget
to addposition (
int
) – the position to insert child in
Insert the child into the self at position.
If a sort function is set, the widget will actually be inserted at the calculated position.
If position is -1, or larger than the total number of items in the self, then the child will be appended to the end.
- invalidate_filter()[source]¶
Update the filtering for all rows.
Call this when result of the filter function on the self is changed due to an external factor. For instance, this would be used if the filter function just looked for a specific search string and the entry with the search string has changed.
- invalidate_headers()[source]¶
Update the separators for all rows.
Call this when result of the header function on the self is changed due to an external factor.
- invalidate_sort()[source]¶
Update the sorting for all rows.
Call this when result of the sort function on the self is changed due to an external factor.
- prepend(child)[source]¶
- Parameters:
child (
Gtk.Widget
) – theGtkWidget
to add
Prepend a widget to the list.
If a sort function is set, the widget will actually be inserted at the calculated position.
- remove(child)[source]¶
- Parameters:
child (
Gtk.Widget
) – the child to remove
Removes a child from self.
- remove_all()[source]¶
Removes all rows from self.
This function does nothing if self is backed by a model.
New in version 4.12.
- select_row(row)[source]¶
- Parameters:
row (
Gtk.ListBoxRow
orNone
) – The row to select
Make row the currently selected row.
- selected_foreach(func, *data)[source]¶
- Parameters:
func (
Gtk.ListBoxForeachFunc
) – the function to call for each selected child
Calls a function for each selected child.
Note that the selection cannot be modified from within this function.
- set_activate_on_single_click(single)[source]¶
- Parameters:
single (
bool
) – a boolean
If single is
True
, rows will be activated when you click on them, otherwise you need to double-click.
- set_adjustment(adjustment)[source]¶
- Parameters:
adjustment (
Gtk.Adjustment
orNone
) – the adjustment
Sets the adjustment (if any) that the widget uses to for vertical scrolling.
For instance, this is used to get the page size for PageUp/Down key handling.
In the normal case when the self is packed inside a
GtkScrolledWindow
the adjustment from that will be picked up automatically, so there is no need to manually do that.
- set_filter_func(filter_func, *user_data)[source]¶
- Parameters:
filter_func (
Gtk.ListBoxFilterFunc
orNone
) – callback that lets you filter which rows to showuser_data (
object
orNone
) – user data passed to filter_func
By setting a filter function on the self one can decide dynamically which of the rows to show.
For instance, to implement a search function on a list that filters the original list to only show the matching rows.
The filter_func will be called for each row after the call, and it will continue to be called each time a row changes (via [method`Gtk`.ListBoxRow.changed]) or when [method`Gtk`.ListBox.invalidate_filter] is called.
Note that using a filter function is incompatible with using a model (see [method`Gtk`.ListBox.bind_model]).
- set_header_func(update_header, *user_data)[source]¶
- Parameters:
update_header (
Gtk.ListBoxUpdateHeaderFunc
orNone
) – callback that lets you add row headersuser_data (
object
orNone
) – user data passed to update_header
Sets a header function.
By setting a header function on the self one can dynamically add headers in front of rows, depending on the contents of the row and its position in the list.
For instance, one could use it to add headers in front of the first item of a new kind, in a list sorted by the kind.
The update_header can look at the current header widget using [method`Gtk`.ListBoxRow.get_header] and either update the state of the widget as needed, or set a new one using [method`Gtk`.ListBoxRow.set_header]. If no header is needed, set the header to
None
.Note that you may get many calls update_header to this for a particular row when e.g. changing things that don’t affect the header. In this case it is important for performance to not blindly replace an existing header with an identical one.
The update_header function will be called for each row after the call, and it will continue to be called each time a row changes (via [method`Gtk`.ListBoxRow.changed]) and when the row before changes (either by [method`Gtk`.ListBoxRow.changed] on the previous row, or when the previous row becomes a different row). It is also called for all rows when [method`Gtk`.ListBox.invalidate_headers] is called.
- set_placeholder(placeholder)[source]¶
- Parameters:
placeholder (
Gtk.Widget
orNone
) – aGtkWidget
Sets the placeholder widget that is shown in the list when it doesn’t display any visible children.
- set_selection_mode(mode)[source]¶
- Parameters:
mode (
Gtk.SelectionMode
) – TheGtkSelectionMode
Sets how selection works in the listbox.
- set_show_separators(show_separators)[source]¶
-
Sets whether the list box should show separators between rows.
- set_sort_func(sort_func, *user_data)[source]¶
- Parameters:
sort_func (
Gtk.ListBoxSortFunc
orNone
) – the sort function
Sets a sort function.
By setting a sort function on the self one can dynamically reorder the rows of the list, based on the contents of the rows.
The sort_func will be called for each row after the call, and will continue to be called each time a row changes (via [method`Gtk`.ListBoxRow.changed]) and when [method`Gtk`.ListBox.invalidate_sort] is called.
Note that using a sort function is incompatible with using a model (see [method`Gtk`.ListBox.bind_model]).
- set_tab_behavior(behavior)[source]¶
- Parameters:
behavior (
Gtk.ListTabBehavior
) – the tab behavior
Sets the behavior of the <kbd>Tab</kbd> and <kbd>Shift</kbd>+<kbd>Tab</kbd> keys.
New in version 4.18.
- unselect_row(row)[source]¶
- Parameters:
row (
Gtk.ListBoxRow
) – the row to unselect
Unselects a single row of self, if the selection mode allows it.
Signal Details¶
- Gtk.ListBox.signals.activate_cursor_row(list_box)¶
- Signal Name:
activate-cursor-row
- Flags:
- Parameters:
list_box (
Gtk.ListBox
) – The object which received the signal
Emitted when the cursor row is activated.
- Gtk.ListBox.signals.move_cursor(list_box, step, count, extend, modify)¶
- Signal Name:
move-cursor
- Flags:
- Parameters:
list_box (
Gtk.ListBox
) – The object which received the signalstep (
Gtk.MovementStep
) – the granularity of the move, as aGtkMovementStep
count (
int
) – the number of step units to moveextend (
bool
) – whether to extend the selectionmodify (
bool
) – whether to modify the selection
Emitted when the user initiates a cursor movement.
The default bindings for this signal come in two variants, the variant with the Shift modifier extends the selection, the variant without the Shift modifier does not. There are too many key combinations to list them all here.
<kbd>←</kbd>, <kbd>→</kbd>, <kbd>↑</kbd>, <kbd>↓</kbd> move by individual children
<kbd>Home</kbd>, <kbd>End</kbd> move to the ends of the box
<kbd>PgUp</kbd>, <kbd>PgDn</kbd> move vertically by pages
- Gtk.ListBox.signals.row_activated(list_box, row)¶
- Signal Name:
row-activated
- Flags:
- Parameters:
list_box (
Gtk.ListBox
) – The object which received the signalrow (
Gtk.ListBoxRow
) – the activated row
Emitted when a row has been activated by the user.
- Gtk.ListBox.signals.row_selected(list_box, row)¶
- Signal Name:
row-selected
- Flags:
- Parameters:
list_box (
Gtk.ListBox
) – The object which received the signalrow (
Gtk.ListBoxRow
orNone
) – the selected row
Emitted when a new row is selected, or (with a
None
row) when the selection is cleared.When the box is using
Gtk.SelectionMode.MULTIPLE
, this signal will not give you the full picture of selection changes, and you should use the [signal`Gtk`.ListBox::selected-rows-changed] signal instead.
- Gtk.ListBox.signals.select_all(list_box)¶
- Signal Name:
select-all
- Flags:
- Parameters:
list_box (
Gtk.ListBox
) – The object which received the signal
Emitted to select all children of the box, if the selection mode permits it.
This is a keybinding signal.
The default binding for this signal is <kbd>Ctrl</kbd>-<kbd>a</kbd>.
- Gtk.ListBox.signals.selected_rows_changed(list_box)¶
- Signal Name:
selected-rows-changed
- Flags:
- Parameters:
list_box (
Gtk.ListBox
) – The object which received the signal
Emitted when the set of selected rows changes.
- Gtk.ListBox.signals.toggle_cursor_row(list_box)¶
- Signal Name:
toggle-cursor-row
- Flags:
- Parameters:
list_box (
Gtk.ListBox
) – The object which received the signal
Emitted when the cursor row is toggled.
The default bindings for this signal is <kbd>Ctrl</kbd>+<kbd>␣</kbd>.
- Gtk.ListBox.signals.unselect_all(list_box)¶
- Signal Name:
unselect-all
- Flags:
- Parameters:
list_box (
Gtk.ListBox
) – The object which received the signal
Emitted to unselect all children of the box, if the selection mode permits it.
This is a keybinding signal.
The default binding for this signal is <kbd>Ctrl</kbd>-<kbd>Shift</kbd>-<kbd>a</kbd>.
Property Details¶
- Gtk.ListBox.props.accept_unpaired_release¶
- Name:
accept-unpaired-release
- Type:
- Default Value:
- Flags:
Whether to accept unpaired release events.
- Gtk.ListBox.props.activate_on_single_click¶
- Name:
activate-on-single-click
- Type:
- Default Value:
- Flags:
Determines whether children can be activated with a single click, or require a double-click.
- Gtk.ListBox.props.selection_mode¶
- Name:
selection-mode
- Type:
- Default Value:
- Flags:
The selection mode used by the list box.
- Gtk.ListBox.props.show_separators¶
- Name:
show-separators
- Type:
- Default Value:
- Flags:
Whether to show separators between rows.
- Gtk.ListBox.props.tab_behavior¶
- Name:
tab-behavior
- Type:
- Default Value:
- Flags:
Behavior of the <kbd>Tab</kbd> key
New in version 4.18.